Integrating chatbot with website-RASA + Flask
Integrating chatbot with website-RASA + Flask

In the previous article, I’ve discussed how to develop a basic chatbot using RASA. This article will focus on integrating the chatbot with a website using Flask.
Once we have our trained chatbot ready, we are ready to integrate the chatbot to a website through Flask. There is more than 1 way to do this and I’ll show you how to do the integration using python.
As we’ll be using Flask, the first step is to install Flask, which can be done using:
```
pip install Flask
```
Once the installation is done, create a file inside the project folder and name it as ‘app.py’. This is where we’ll have our backend program.
```
from flask import Flask, render_template, request, jsonify
import os,sys,requests, json
from random import randint
app = Flask(__name__,template_folder='templates'
)
@app.route('/')
def home():
return render_template('index.html')
@app.route('/parse',methods=['POST', 'GET'] )
def extract():
text=str(request.form.get('value1'))
payload = json.dumps({"sender": "Rasa","message": text})
headers = {'Content-type': 'application/json', 'Accept': 'text/plain'}
response = requests.request("POST", url="http://localhost:5005/webhooks/rest/webhook", headers=headers, data=payload)
response=response.json()
resp=[]
for i in range(len(response)):
try:
resp.append(response[i]['text'])
except:
continue
result=resp
return render_template('index.html', result=result,text=text)
if __name__ == "__main__":
app.run(debug=True)
```
The working of the code is pretty straightforward. We begin by importing the necessary libraries and create a Flask object called ‘app’. Then we redirect our homepage to index.html. That’s where we’ll have the HTML code for the front-end.
Next, we’ll add an endpoint with POST and GET methods. Inside the extract function, we get the user input message using request.form.get(). Then, we’ll convert the input text into JSON format and pass the data to our chatbot server which will be running at the default port 5005. Our chatbot processes the data and sends the response which we’ll convert to JSON format. Then, we’ll send the response from our chatbot back to the front-end (index.html), along with the user’s input.
To get the website working, firstly, we have to start the chatbot as a server. Navigate to the project folder in the terminal and run the following command:
```
rasa run -m models --enable-api
#or
rasa run -m models --enable-api cors "*"
```
Then open a new terminal, navigate to the folder and start the web application using:
```
python app.py
#or
python3 app.py
```
Now click on the localhost and you should be able to communicate with the chatbot from the front-end !!!
Solutions of “jinja2.exceptions.TemplateNotFound: index.html”
While runing FLASK_APP=app.py FLASK_DEBUG=true flask run in the command line, sometimes you may see a TemplateNotFound Exception e.g. “jinja2.exceptions.TemplateNotFound: index”. Here is a step-by-step guide that will walk you through how you may have this issue resolved.
The error message was due to the lack of a “index.html” file in the “Template” directory. To resolve the issue, simply create a folder name it “Templates”. Then move “index.html” into this newly created folder.
Besides, in file “app.py”, revisions need to be made so that it reads something like this:
app=Flask(__name__,template_folder='templates')
Save this. Flask runs again and there wouldn’t be any error this time!
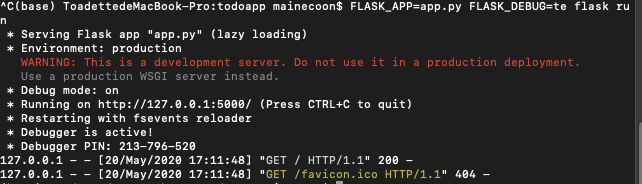

References:
Author - Ajaykumaar S
Website 1 - https://sites.miis.edu/dreadkingrathalos/2020/05/20/solutions-of-jinja2-exceptions-templatenotfound-index-html/
website 2 - https://medium.com/featurepreneur/integrating-chatbot-with-website-rasa-flask-4569f18d31be
Comments
Post a Comment