Python | ToDo webapp using Django
Prerequisite : django installation
Django
is a high-level Python Web framework based web framework that allows
rapid development and clean, pragmatic design. today we will create a
todo app created to understand the basics of Django. In this web app,
one can create notes like Google Keep or Evernote.
Modules required :
pip install --upgrade django-crispy-forms
basic setup :
Start a project by the following command –
django-admin startproject todo-site
Change directory to todo-site –
cd todo-site
Start the server- Start the server by typing following command in terminal –
python manage.py runserver
To check whether the server is running or not go to a web browser and enter http://127.0.0.1:8000/ as URL.
Now stop the server by pressing
ctrl-c
Let’s create an app now.
python manage.py startapp todo
Goto todo/ folder by doing : cd todo and create a folder with index.html file : templates/todo/index.html
Open the project folder using a text editor. The directory structure should look like this :
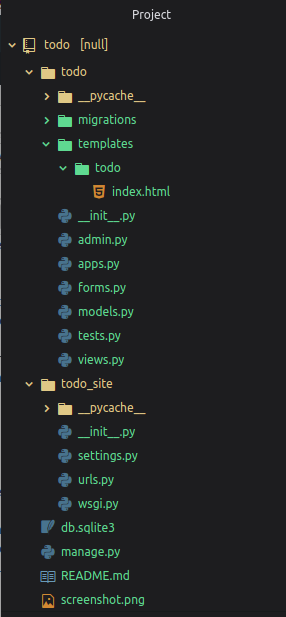
Now add todo app and crispty_form in your todo_site in settings.py.
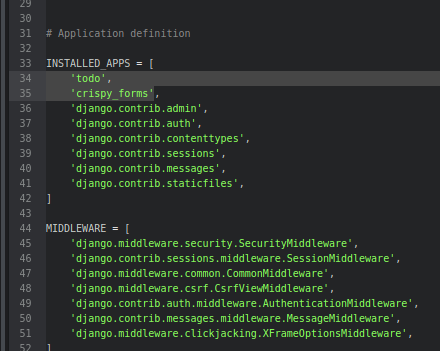
Edit urls.py file in todo_site :
from django.contrib import admin
from django.urls import path
from todo import views
urlpatterns = [
path('', views.index, name = "todo" ),
path( 'del/<str:item_id>' , views.remove, name = "del" ),
path( 'admin/' , admin.site.urls),
]
|
Edit models.py in todo :
from django.db import models
from django.utils import timezone
class Todo(models.Model):
title = models.CharField(max_length = 100 )
details = models.TextField()
date = models.DateTimeField(default = timezone.now)
def __str__( self ):
return self .title
|
Edit views.py in todo :
from django.shortcuts import render, redirect
from django.contrib import messages
from .forms import TodoForm
from .models import Todo
def index(request):
item_list = Todo.objects.order_by( "-date" )
if request.method = = "POST" :
form = TodoForm(request.POST)
if form.is_valid():
form.save()
return redirect( 'todo' )
form = TodoForm()
page = {
"forms" : form,
"list" : item_list,
"title" : "TODO LIST" ,
}
return render(request, 'todo/index.html' , page)
def remove(request, item_id):
item = Todo.objects.get( id = item_id)
item.delete()
messages.info(request, "item removed !!!" )
return redirect( 'todo' )
|
Now create a forms.py in todo :
from django import forms
from .models import Todo
class TodoForm(forms.ModelForm):
class Meta:
model = Todo
fields = "__all__"
|
Register models to admin :
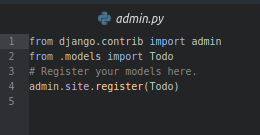
Navigate to templates/todo/index.html and edit it : link to index.html file
Make migrations and migrate it
python manage.py makemigrations
python manage.py migrate
Now you can run the server to see your todo app
python manage.py runserver
index.html
```
</html>````
if you are facing an error templtes not found then please refer below
Find this tuple:
import os
SETTINGS_PATH = os.path.dirname(os.path.dirname(__file__))
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
You need to add to 'DIRS' the string
os.path.join(SETTINGS_PATH, 'templates')
So altogether you need:
import os
SETTINGS_PATH = os.path.dirname(os.path.dirname(__file__))
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [os.path.join(SETTINGS_PATH, 'mysite/templates')],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
complete source code - https://github.com/rabiyulfahimhasim786/django_resources_/tree/main/django-todoapp-site/mysite
reference- geeksforgeeks.org
Comments
Post a Comment