Create Web Based ChatBot in Python, Django, Flask
Create Web Based ChatBot in Python, Django, Flask
Learn how to create Chatbot in Python. I this tutorial, we will use Chatterbot Library for creating the chat bot. We will use Flask Framework for deploying the chatbot on web. This tutorial change be used with Django also.
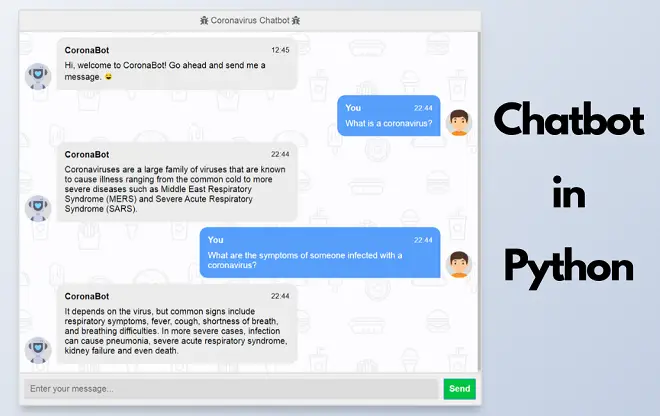
Every Chatbot has a theme. Our chatbot is going to Answer the Questions of User of Coronavirus Disease. It will greet the user, interact with them and give answers of Covid-19.
Installing Chatbot Required Libraries
All the libraries are installed in Virtualenv.
pip install Flask
pip install chatterbot
pip install chatterbot-corpus
Create Chatbot in Python
Now we have ca chatterbot, we will create a chatbot.py
file and paste the bellow code.
from chatterbot import ChatBot from chatterbot.trainers import ListTrainer from chatterbot.trainers import ChatterBotCorpusTrainer # Creating ChatBot Instance chatbot = ChatBot('CoronaBot') # Training with Personal Ques & Ans conversation = [ "Hello", "Hi there!", "How are you doing?", "I'm doing great.", "That is good to hear", "Thank you.", "You're welcome." ] trainer = ListTrainer(chatbot) trainer.train(conversation) # Training with English Corpus Data trainer_corpus = ChatterBotCorpusTrainer(chatbot) trainer_corpus.train( 'chatterbot.corpus.english' )
In the above code, we are creating a chatterbot instance. Then we are training our chatbot with ListTrainer with our personal question and answers. Then we are using chatterbot corpus english data to train our chatbot.
Creating Web UI for Chatbot using Flask
As we have installed Flask, we will create a app.py
file and paste the bellow code inside it.
from chatbot import chatbot from flask import Flask, render_template, request app = Flask(__name__) app.static_folder = 'static' @app.route("/") def home(): return render_template("index.html") @app.route("/get") def get_bot_response(): userText = request.args.get('msg') return str(chatbot.get_response(userText)) if __name__ == "__main__": app.run()
In the above code, we are creating routes for our web application. In get_bot_response()
we are taking input from html form and after processing chatbot giving response.
HTML Template for ChatBot
Create a folder templates
and add this index.html inside it – Download.
Create a folder static
, inside it create styles
folder and inside it add this file style.css
– Download
The above file path should match like bellow –
D:/chatbot/static/styles/style.css D:/chatbot/templates/index.html
In index.html
file, we have written a script
for sending the data which is input to chatbot form and take the response to append it to chatbot window.
Running Chatbot Project
Once the you have setup the project, we need to just run the flask app.
python app.py
If everything goes right, go to – http://localhost:5000/ and enjoy your own personal chatbot.
Note – If you see not see right answer for question, delete the .sqlite3
database file from your folder.
Optimizing and Adding Feature to Chatbot
We have created chatbot successfully, but it as basic example. We need to add more features to our chatbot.
Open your chatbot.py
file and do some changes like
Replace
chatbot = ChatBot('CoronaBot',)
with
chatbot = ChatBot( 'CoronaBot', storage_adapter='chatterbot.storage.SQLStorageAdapter', logic_adapters=[ 'chatterbot.logic.MathematicalEvaluation', 'chatterbot.logic.TimeLogicAdapter', 'chatterbot.logic.BestMatch', { 'import_path': 'chatterbot.logic.BestMatch', 'default_response': 'I am sorry, but I do not understand. I am still learning.', 'maximum_similarity_threshold': 0.90 } ], database_uri='sqlite:///database.sqlite3' )
In above replacing, we have added default answer, database and added logic adapter which can answer maths and time questions.
Using file for question and answer
In the above, we have used conversation list. Now we will replace with it a file where question and answer are written inside it are loaded and fed to ListTrainer.
Replace
# Training with Personal Ques & Ans conversation = [ "Hello", "Hi there!", "How are you doing?", "I'm doing great.", "That is good to hear", "Thank you.", "You're welcome." ] trainer = ListTrainer(chatbot) trainer.train(conversation)
with
training_data_quesans = open('training_data/ques_ans.txt').read().splitlines() training_data_personal = open('training_data/personal_ques.txt').read().splitlines() training_data = training_data_quesans + training_data_personal trainer = ListTrainer(chatbot) trainer.train(training_data)
We are loading data form training_data/ques_ans.txt
and training_data/personal_ques.txt
. You can download those files from here.
Project Github
Tags: chatbot, chatterbot, flask
Comments
Post a Comment